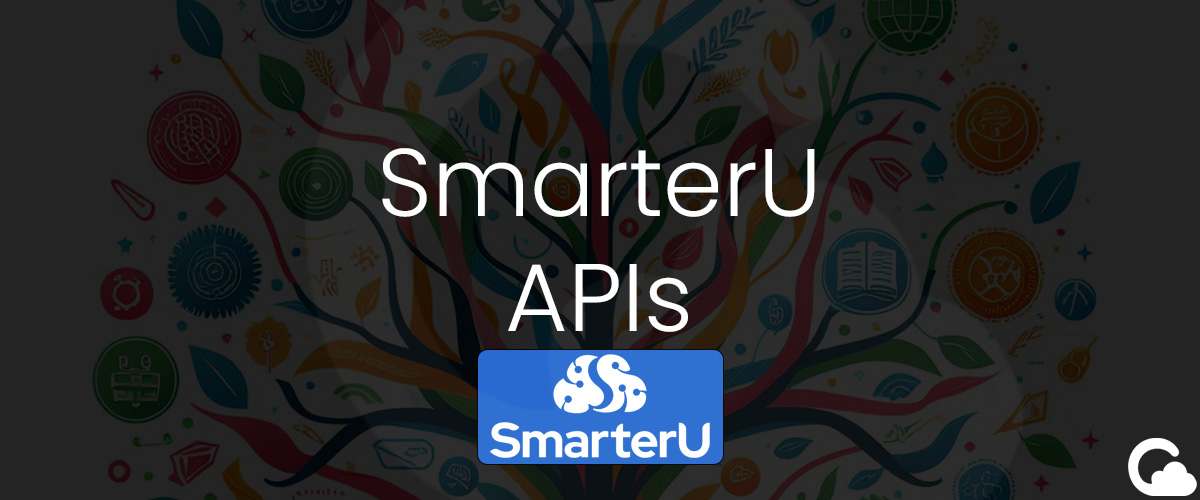
SmarterU - Utilizing APIs - Postman & Python
This week we worked on an integration with SmarterU APIs. The APIs for SmarterU library gives you an idea of all the different types of APIs you can call, but does not describe how to query them. Through trial and error, we were able to send our first successful API call. This blogs intention is to make it easier for developers in the future to understand what structure SmarterU APIs are expecting.
Problem
Without an actual explanation or Postman example library, there is a lot of guess work to begin querying APIs from SmarterU. The APIs appear to be a second version (v2), but don't utilize standard endpoint calls for APIs and require all requests to be POST HTTPS requests. Other than this basic information, it would be easier if they had an example or library to utilize.
Solution
1. Begin by logging in as an Administrator to your SmarterU tenant (i.e. https://tenant.smarteru.com).
2. Navigate to Account Settings by going to Account Admin > Account Settings.
Enable API access & AccountAPI Key
3. Scroll to API Setup and expand this section.
4. Check off Allow API access for this account > Scroll back to the top and click Save. (Optional: You can use API Allowed Sources if you want to only allow API calls from specific IP sources).
5. In the API Setup section you will see API Key. You will need to store this for later. We will identify this key as AccountAPI.
Generate UserAPI Key
Next, we will need to generate the UserAPI. You can either use your Admin account, or you can create a new user specifically for API calls. It may be wise to create a new user account that has admin as the account won't be affected if you leave the organization.
6. Navigate to User Admin > Users > find the account you want to generate the UserAPI Key with and hit Edit.
7. Under Login Information > Check off the box Enable API and a new API Key will be generated. Scroll up and click Save You will need this API Key for later as we will identify this key as UserAPI.
Postman Call
The APIs for SmarterU provide you the XML structure of the API call, but the most important piece is to understand is how to make the call in Postman.
8. Start off by logging into Postman.
9. Next, start off by creating a new Workspace in Postman for your SmarterU collection.
10. Navigate to Environments and you can either set it in Globals or create a new Environment with the AccountAPI key and UserAPI key.

11. Navigate to Collections and click New. After creating the collection, right click your Collection and click New Request
12. Change the request type to POST and enter https://api.smarteru.com/apiv2/
13. Go to Body > choose x-www-form-urlencoded > enter Package under they Key column and use the following XML structure in Value:
<SmarterU>
<AccountAPI>{{AccountAPI}}</AccountAPI>
<UserAPI>{{UserAPI}}</UserAPI>
<Method>getLearnerCredentialReport</Method>
<Parameters>
<Report>
<Type>DetailedList</Type>
<Page>
<Num>1</Num>
<Size>100</Size>
</Page>
<Filters>
<Status>Accepted</Status>
</Filters>
</Report>
</Parameters>
</SmarterU>
More specifically, this API call uses getLearnerCredentialReport and calls the first page (100 rows) with the Status being filtered by Accepted only. Here is a demonstration of the API call in Postman:
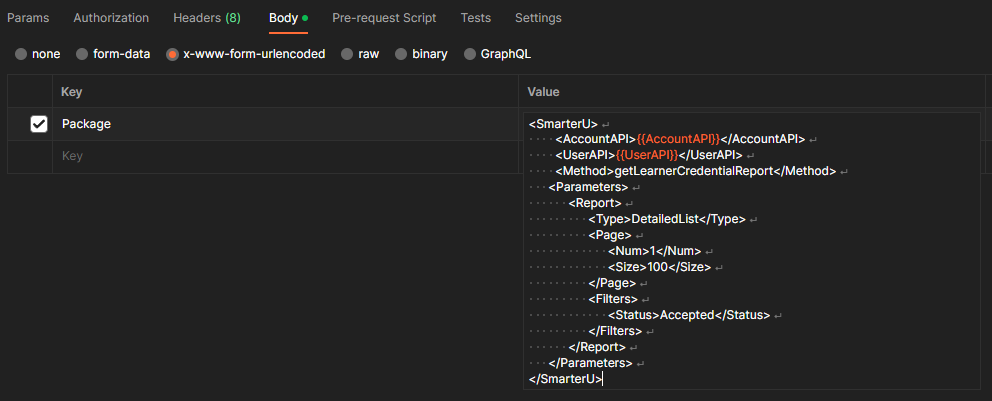
You can also download the Postman Example here.
Using Python to Call SmarterU APIs
Now that you are more familiar with SmarterU APIs you can utilize the following Python code to call SmarterU APIs:
import requests
URL = "https://api.smarteru.com/apiv2/"
# Ensure you add AccountAPI and UserAPI to the XML call in PARAMS
PARAMS = {
"Package": """<SmarterU>
<AccountAPI>YourAccountAPIKey</AccountAPI>
<UserAPI>YourUserAPIKey</UserAPI>
<Method>getLearnerCredentialReport</Method>
<Parameters>
<Report>
<Type>DetailedList</Type>
<Page>
<Num>1</Num>
<Size>100</Size>
</Page>
<Filters>
<Status>Accepted</Status>
</Filters>
</Report>
</Parameters>
</SmarterU>
"""
}
HEADERS = {'content-type': 'application/x-www-form-urlencoded'}
r = requests.post(url = URL, data = PARAMS, headers = HEADERS)
print (r.text)
Summary
As the SmarterU API library is not overly intiutive, this blog gives you a great starting point and overview of how to query their APIs. Hopefully you have a good foundation for making calls with both Postman and Python going forward. Perhaps at some point, we can release a full Postman collection for SmarterU if there is enough interest generated.