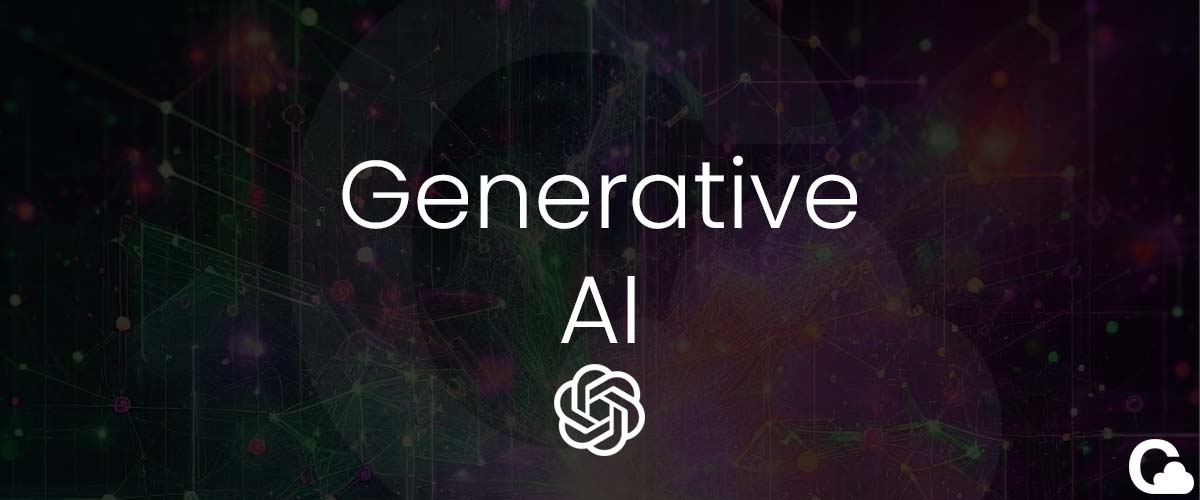
Leveraging Multi-Agent Frameworks and Advanced Prompting Techniques in Healthcare AI
In the rapidly evolving field of artificial intelligence, multi-agent frameworks and sophisticated prompting techniques are revolutionizing how we build intelligent systems. This blog post will explore how to implement these concepts using the OpenAI and Anthropic APIs, with a focus on creating a healthcare assistant agent.
Multi-Agent Frameworks
Multi-agent frameworks involve the use of multiple AI agents working together to solve complex problems. Each agent can have specialized knowledge or capabilities, allowing for more robust and versatile AI systems.
Example: Healthcare Assistant Multi-Agent System
Let's create a simple multi-agent system for a healthcare assistant using both OpenAI and Anthropic APIs. We'll have three agents:
- Translator Agent (OpenAI)
- Healthcare Advisor Agent (Anthropic)
- Response Evaluator Agent (OpenAI)
Here's how we can implement this system:
import openai
from anthropic import Anthropic
# Initialize clients
openai_client = openai.OpenAI(api_key="your_openai_api_key")
anthropic_client = Anthropic(api_key="your_anthropic_api_key")
# Functions defined for each agent...
This multi-agent system translates the query if needed, provides healthcare advice, and then evaluates the quality of the advice.
Advanced Prompting Techniques
To enhance the performance of our AI agents, we can employ various prompting techniques:
1. Chain-of-Thought (CoT) Prompting
CoT prompting encourages the model to break down complex problems into step-by-step reasoning. Here's how we can modify our healthcare advisor agent to use CoT:
def cot_healthcare_advisor_agent(query):
prompt = f"""As a healthcare advisor, provide advice for the following query. Use a step-by-step approach to reason through your response.
Query: {query}
Step 1: Understand the query
Step 2: Identify key health concerns
Step 3: Consider relevant medical information
Step 4: Formulate advice
Step 5: Provide a concise summary
Advice:"""
response = anthropic_client.completions.create(
model="claude-2",
prompt=prompt,
max_tokens_to_sample=500
)
return response.completion.strip()
2. ReAct (Reasoning and Acting) Prompting
ReAct combines reasoning with action steps. We can implement this for our evaluator agent:
def react_evaluator_agent(original_query, response):
prompt = f"""Evaluate the following healthcare advice for accuracy, completeness, and clarity. Use the ReAct (Reasoning and Acting) framework to structure your evaluation.
Original Query: {original_query}
Healthcare Advice: {response}
Thought 1: Consider the accuracy of the advice
Action 1: Check if the advice aligns with current medical knowledge
Observation 1: [Your observation here]
Thought 2: Assess the completeness of the response
Action 2: Identify any missing crucial information
Observation 2: [Your observation here]
Thought 3: Evaluate the clarity of the advice
Action 3: Determine if the advice is easy to understand for a general audience
Observation 3: [Your observation here]
Thought 4: Summarize the evaluation
Action 4: Provide a concise evaluation summary
Observation 4: [Your observation here]
Evaluation Summary:"""
response = openai_client.chat.completions.create(
model="gpt-4",
messages=[{"role": "user", "content": prompt}]
)
return response.choices[0].message.content.strip()
3. Few-Shot Prompting
Few-shot prompting provides examples to guide the model's responses. Here's how we can use it with our translator agent:
def few_shot_translator_agent(text, target_language="en"):
prompt = f"""Translate the following text to {target_language}. If it's already in {target_language}, return it as is.
Examples:
English: "Hello, how are you?"
Spanish: "Hola, ¿cómo estás?"
Spanish: "¿Dónde está el hospital?"
English: "Where is the hospital?"
Text to translate: {text}
Translation:"""
response = openai_client.chat.completions.create(
model="gpt-3.5-turbo",
messages=[{"role": "user", "content": prompt}]
)
return response.choices[0].message.content.strip()
Conclusion
By combining multi-agent frameworks with advanced prompting techniques, we can create more sophisticated and effective AI systems. In our healthcare assistant example, we've demonstrated how different agents can work together to provide translated, accurate, and evaluated healthcare advice. The use of techniques like Chain-of-Thought, ReAct, and Few-Shot prompting further enhances the quality and reliability of our AI-generated responses.
As you implement these concepts, remember to continuously refine your prompts and agent interactions based on the specific requirements of your healthcare application. With the power of OpenAI and Anthropic's advanced language models, the possibilities for creating intelligent and helpful AI systems are truly exciting.
REF: Full end-to-end agent
Here's a complete example of a healthcare assistant using few-shot prompting for the translator agent:
import openai
from anthropic import Anthropic
# Initialize clients
openai_client = openai.OpenAI(api_key="your_openai_api_key")
anthropic_client = Anthropic(api_key="your_anthropic_api_key")
def few_shot_translator_agent(text, target_language="en"):
prompt = f"""Translate the following text to {target_language}. If it's already in {target_language}, return it as is.
Examples:
English: "Hello, how are you?"
Spanish: "Hola, ¿cómo estás?"
Spanish: "¿Dónde está el hospital?"
English: "Where is the hospital?"
Text to translate: {text}
Translation:"""
response = openai_client.chat.completions.create(
model="gpt-3.5-turbo",
messages=[{"role": "user", "content": prompt}]
)
return response.choices[0].message.content.strip()
def cot_healthcare_advisor_agent(query):
prompt = f"""As a healthcare advisor, provide advice for the following query. Use a step-by-step approach to reason through your response.
Query: {query}
Step 1: Understand the query
Step 2: Identify key health concerns
Step 3: Consider relevant medical information
Step 4: Formulate advice
Step 5: Provide a concise summary
Advice:"""
response = anthropic_client.completions.create(
model="claude-2",
prompt=prompt,
max_tokens_to_sample=500
)
return response.completion.strip()
def react_evaluator_agent(original_query, response):
prompt = f"""Evaluate the following healthcare advice for accuracy, completeness, and clarity. Use the ReAct (Reasoning and Acting) framework to structure your evaluation.
Original Query: {original_query}
Healthcare Advice: {response}
Thought 1: Consider the accuracy of the advice
Action 1: Check if the advice aligns with current medical knowledge
Observation 1: [Your observation here]
Thought 2: Assess the completeness of the response
Action 2: Identify any missing crucial information
Observation 2: [Your observation here]
Thought 3: Evaluate the clarity of the advice
Action 3: Determine if the advice is easy to understand for a general audience
Observation 3: [Your observation here]
Thought 4: Summarize the evaluation
Action 4: Provide a concise evaluation summary
Observation 4: [Your observation here]
Evaluation Summary:"""
response = openai_client.chat.completions.create(
model="gpt-4",
messages=[{"role": "user", "content": prompt}]
)
return response.choices[0].message.content.strip()
def healthcare_assistant(user_query, language="en"):
# Step 1: Translate the query if needed
translated_query = few_shot_translator_agent(user_query, target_language="en")
# Step 2: Get healthcare advice
healthcare_advice = cot_healthcare_advisor_agent(translated_query)
# Step 3: Evaluate the response
evaluation = react_evaluator_agent(translated_query, healthcare_advice)
# Step 4: Translate the advice back to the original language if needed
if language != "en":
healthcare_advice = few_shot_translator_agent(healthcare_advice, target_language=language)
evaluation = few_shot_translator_agent(evaluation, target_language=language)
return {
"original_query": user_query,
"translated_query": translated_query,
"healthcare_advice": healthcare_advice,
"evaluation": evaluation
}
# Example usage
user_query = "What are the symptoms of the flu?"
result = healthcare_assistant(user_query)
print(f"Original Query: {result['original_query']}")
print(f"Translated Query: {result['translated_query']}")
print(f"Healthcare Advice: {result['healthcare_advice']}")
print(f"Evaluation: {result['evaluation']}")