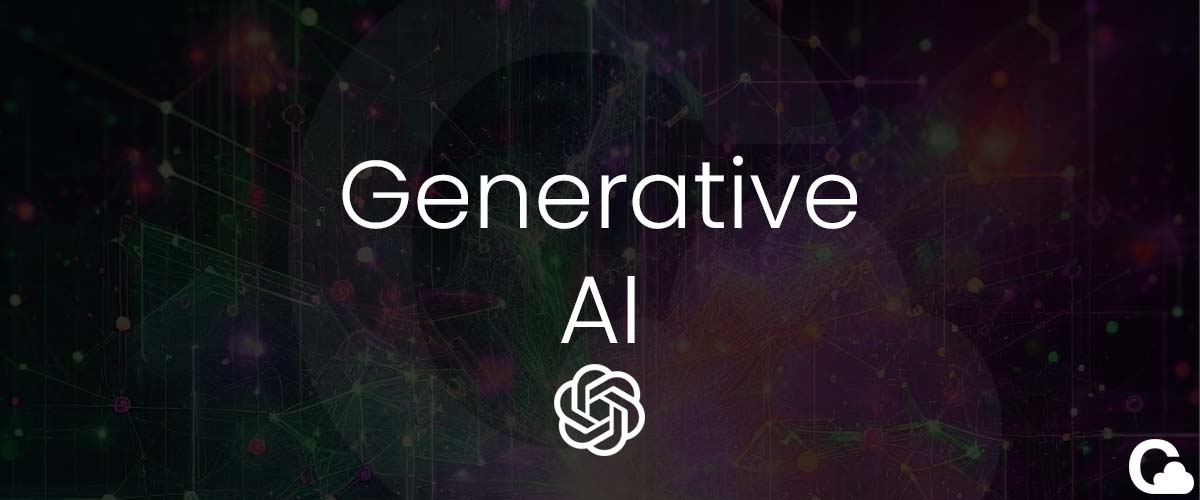
How to Fine-Tune Llama 3.1 using Google Colab
What is Llama 3.1?
Meta's Llama 3.1 is the latest open-source large language model, boasting an impressive 405 billion parameters and multilingual capabilities. This model has set a new standard in AI accessibility, making advanced language processing tools available to a broader audience. Key features include:
- Massive Scale: 405 billion parameters, trained on over 15 trillion tokens with 16,000+ Nvidia H100 GPUs.
- Open-Source Accessibility: Developers can download, customize, and deploy the model.
- Multilingual Support: Capable of processing 8 languages, including English, German, French, Italian, Portuguese, Hindi, Spanish, and Thai.
- Extended Context Window: A 128k token context window, useful for long documents and conversations.
- High Performance: Comparable with leading proprietary models like GPT-4 and Claude 3.5 Sonnet.
- Cost-Efficiency: Lower running costs, estimated at half that of its competitors.
- Built-In Safeguards: Features to mitigate harmful outputs while allowing for customization.
These attributes make Llama 3.1 a versatile tool for various AI applications, such as multilingual chatbots and advanced coding assistants.
Fine-Tuning on Google Colab
Fine-tuning Llama 3.1 8B on Google Colab involves several steps. Here’s a comprehensive guide to help you through the process:
Step-by-Step Guide
1. Setting Up the Environment
First, you need to set up a new notebook in Google Colab and enable GPU runtime to leverage the computational power required for fine-tuning.
# Set up environment
!pip install unsloth[cu118] -U
!pip install accelerate
!pip install bitsandbytes
2. Loading the Pre-Quantized Model
Using the Unsloth library, load the pre-quantized Llama 3.1 8B model, which helps in optimizing memory usage.
from unsloth import FastLanguageModel
import torch
# Load the pre-quantized model
model, tokenizer = FastLanguageModel.from_pretrained(
"unsloth/llama-3.1-8b-instruct",
max_seq_length=2048,
dtype=None,
load_in_4bit=True,
)
3. Preparing Your Custom Dataset
Prepare your dataset in a compatible format like JSONL. For this example, we’ll use a sample dataset from the Hugging Face Datasets library.
from datasets import load_dataset
# Load dataset
dataset = load_dataset("vicgalle/alpaca-gpt4")
4. Defining Training Arguments
Set up training arguments such as epochs, batch size, and learning rate.
from transformers import TrainingArguments
# Set up training arguments
training_args = TrainingArguments(
output_dir="./results",
num_train_epochs=3,
per_device_train_batch_size=4,
learning_rate=2e-4,
fp16=True,
)
5. Initializing the Trainer
Initialize the SFTTrainer with the model, dataset, and training parameters.
from trl import SFTTrainer
# Initialize trainer
trainer = SFTTrainer(
model=model,
args=training_args,
train_dataset=dataset["train"],
dataset_text_field="text",
)
6. Starting the Fine-Tuning Process
Begin the fine-tuning process.
# Start fine-tuning
trainer.train()
7. Saving the Fine-Tuned Model
After fine-tuning, save the model for later use.
# Save the fine-tuned model
trainer.save_model("./fine_tuned_model")
Saving and Loading Model
To save the fine-tuned model, use the save_pretrained
method. This stores all necessary files, including weights, configuration, and tokenizer, in a specified directory.
# Save model
trainer.save_model("./fine_tuned_model")
To load the saved model for inference, use the from_pretrained
method from the AutoModelForCausalLM
and AutoTokenizer
classes.
from transformers import AutoModelForCausalLM, AutoTokenizer
# Load model
model_path = "./fine_tuned_model"
model = AutoModelForCausalLM.from_pretrained(model_path)
tokenizer = AutoTokenizer.from_pretrained(model_path)
Performing Inference
Inference with the fine-tuned Llama 3.1 8B model can be efficiently performed using the pipeline function from the transformers library.
from transformers import pipeline
# Create text generation pipeline
generator = pipeline("text-generation", model=model, tokenizer=tokenizer)
# Perform inference
prompt = "Explain the concept of machine learning in simple terms."
output = generator(prompt, max_length=200, num_return_sequences=1)
print(output[0]['generated_text'])
This code sets up the model for generating text based on a given prompt. Adjust parameters like max_length
and num_return_sequences
as needed for your specific use case.
Additional Considerations
When loading the model, ensure it is set to evaluation mode with model.eval()
to set dropout and batch normalization layers to evaluation mode, ensuring consistent inference results.
By following this guide, developers and researchers can leverage the power of Llama 3.1 on Google Colab, making advanced AI technology more accessible and customizable.