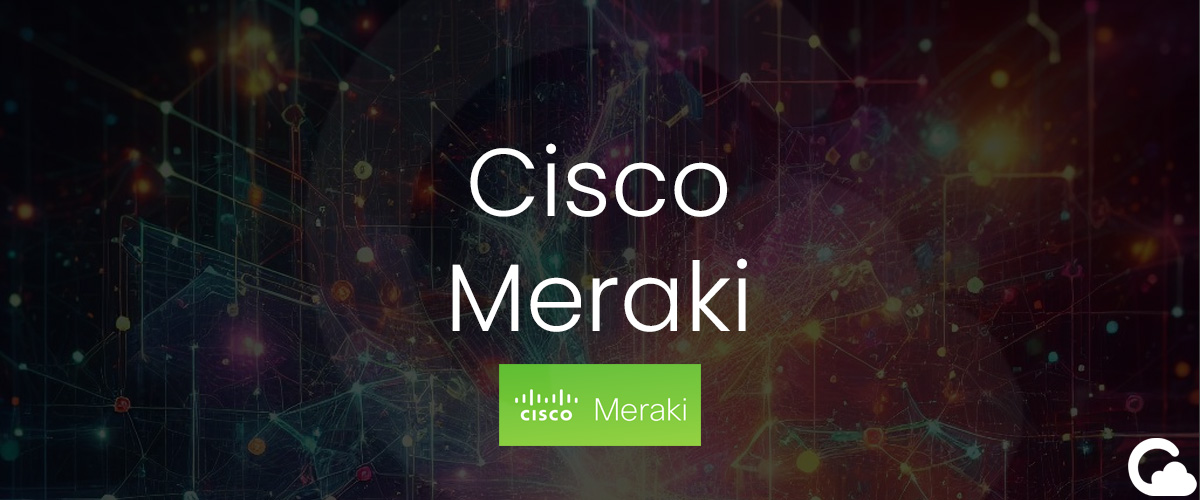
Cisco Meraki - Change Log - Automate Guest Changes and Authorizations Reports with Cisco Meraki APIs and PowerShell
Continuing from last week's requirement of needing a Guest Wi-Fi solution with Guest Ambassadors (click here for blog) a new requirement had been requested. The customer wanted the ability to see who made changes (including creation of user accounts and who authorized Wi-Fi to users). I thought to myself: "it must be possible through Cisco Meraki UI".
Of course it was possible to view this information through the Change Log under Organization (on the Cisco Meraki Dashboard), but how can it be automated? Alerts was the first place I looked, but I then thought: "Meraki must have APIs". After some research and development I came across a solution to query a Cisco Meraki's organization-specific data and report these types of events.
Problem
How does a person automate reports from Cisco Meraki that includes Change Log information and more specifically, who created Guest Accounts and authorized access to Wi-Fi?
Solution
1. First, start by logging into your Cisco Meraki (Cloud) dashboard at https://account.meraki.com/login/dashboard_login
2. Next, select your Organization that you want to automate reports for.
3. On the left-hand menu, select Organization > Settings.
4. Scroll down to Dashboard API Access and make sure Enable access to the Cisco Meraki Dashboard API is checked (enabled). Then click Save Changes.
Generate API Key
5. On the left-hand menu, select Organization > API & webhooks.
6. Press the Generate API Key button > Copy your API Key > Check off I've stored my API key > Press Done. Make sure you save this API key somewhere as we will need it to make the API calls. Note: You can only have two API keys generated at once and you must delete one (revoke) if you want to generate a new key.
Find your Organization ID
7. The easiest place to find it is by scrolling to the bottom of any Cisco Meraki Dashboard page. It should state something like "Data for SMART-SERVICES-CLOUDAEN (organization ID: 111111111111111111) is hosted in North America". Example:

PowerShell - Cisco Meraki API
8. Open your favourite PowerShell editor (i.e. Visual Studio or PowerShell ISE) and input the following script:
# Enter your Organization ID & API Key
$organizationId = "111111111111111111"
$apiKey = "Enter API Key Here"
$headers = @{
Authorization="Bearer $apiKey"
Content='application/json'
}
$return = Invoke-RestMethod -Method Get -Uri "https://api.meraki.com/api/v1/organizations/$organizationId/configurationChanges?timespan=604800" -Headers $headers
Notes: You will need your Organization ID for $organizationId, and your API Key for $apiKey. In the $return, you can add the timespan. I just used 604800 (which is in seconds, so it is equivalent to 7 days). The 604800 will return the last 7 days of data from the current date of the API call.
9. Now that we have the information stored in $return we can now use PowerShell's Where-Object to extract data using the following PowerShell example:
# Enter your information below to determine the location of the CSV
$return | Where-Object { $_.page -eq "Users" } | export-csv "C:\temp\changeLogs.csv"
Notes: The Where-Object is specifically checking for information related to "Users" which is user changes. If you want to check for other types of logs, just run another command $return and it will give you a full response with other page types.
Full PowerShell Script
# Enter your Organization ID & API Key
$organizationId = "111111111111111111"
$apiKey = "Enter API Key Here"
$headers = @{
Authorization="Bearer $apiKey"
Content='application/json'
}
$return = Invoke-RestMethod -Method Get -Uri "https://api.meraki.com/api/v1/organizations/$organizationId/configurationChanges?timespan=604800" -Headers $headers
# Enter where you would like the CSV to export to
$return | Where-Object { $_.page -eq "Users" } | export-csv "C:\temp\changeLogs.csv"
If generating a CSV isn't enough, you can send e-mails with PowerShell's Send-MailMessage but for now we are just generating a log file. Perhaps in another blog we can explain how to generate an e-mail within Office 365 using Enterprise Applications, certificates and OAUTH 2.0.
Sample Output

The most important piece is the oldValue and newValue. Just examining the output we can see:
{"User description":"Test","User email":"[email protected]","User password":"password hidden","Account type":"guest","User authorized":"true","Authorized by":"[email protected]","Expires at":"2023-11-22 21:45:24 UTC"}
This log tells us that the User "Test" with the e-mail "[email protected]" who is a Guest was authorized by [email protected] to have access until 2023-11-22 21:45:24 UTC. Therefore, if I were to e-mail this log once a day or weekly, the customer would then know who has been granting access and which Guests have had the ability to access the network.
Summary
As customers are becoming more aware of the benefits of technology and reporting, more complex requirements are being generated. Vendors such as Cisco have been responding to these types of needs by building APIs which allow customers to access their data and automate the outputs. Cisco Meraki (Cloud), offers a robust API library for finding Organizational data with regards to their networks. We can use this data output to craft reports and automate getting this information to whomever requires it.
Related Documentation
Cisco Meraki: API Introduction
Cisco Meraki: API Authentication